Building a Unity3d game I needed to connect to an Arduino controller. My first approach was to go to the Unity3d asset store and download a Bluetooth plugin for Unity3d. You will find some good ones that support IOS and android, they do cost some money.
When it came time to launch the game and play the game with the controller. In order for the plugin to work, you have to add this line to your AndroidManifest.xml.
<uses-permission android:name=”android.permission.ACCESS_COARSE_LOCATION” />
Adding this line, On installation and the first launch of the game, you will be prompted with this dialog!
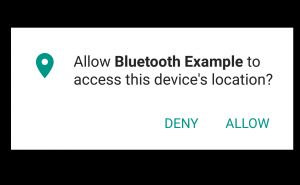
This wasn’t going to work, most people will push deny (I know I do). Any direct Bluetooth communication to your game needs this permission, and I didn’t want to add this friction to the game.
Wanting to do basic user input of Arduino buttons to a mobile game. I realized that store-bought Bluetooth gamepads for works with the phone without these warnings. How do gamepads work in a Unity3d game with no plugin?
After a few hours of research, I discovered the answer. HID Human Interface Device. This is the profile your Bluetooth keyboards, mouse and gamepads use to communicate to the mobile device. Most Bluetooth LE microcontroller supports this profile.
Using this profile you have all the keys on the keyboard available to you to send to your device. Here are the keycodes that unity3d supports. https://docs.unity3d.com/ScriptReference/KeyCode.html
The only code you need in the game is Input.GetKeyUp() Input.GetKeyDown() in an update() loop.
void Update () { if (SupportedKeysDown() == true ) { // your response here } } private bool SupportedKeysUp() { return Input.GetKeyUp(KeyCode.Keypad0) || Input.GetKeyUp(KeyCode.F1 ); } private bool SupportedKeysDown() { return Input.GetKeyDown(KeyCode.Keypad0) || Input.GetKeyUp(KeyCode.F1); }
Pros
- Native Unity3d support of your controller on Android and IOS. When using a Bluetooth plugin it is up to the developer and user to connect the controller to a game. All you have to do is pair your Bluetooth controller to your device in the setting menu and you are done.
- It is free! Most of the good Bluetooth plugins for Unity3d cost money.
- When testing your game you are able to simulate the Bluetooth controller in the Unity3d editor environment by using the keyboard.
Cons
- Use keys like Numpads and F1 Keys. Don’t use a keycode that the Android or IOS phone keypad/keyboards use. For example a letter of the alphabet. Your controller is now a keyboard to your device. When you leave your game, the controller will send keyboard events that register to the phone’s environments.
If you want Arduino Bluetooth code for your microcontroller. Please visit: https://github.com/3lbsadmin/CycleVR/wiki
Helpful Links and Notes from my research:
https://www.bluetooth.com/specifications/adopted-specifications
https://github.com/djnugent/BLE_WASD
https://forums.adafruit.com/viewtopic.php?f=22&t=93409&start=15
https://forums.adafruit.com/viewtopic.php?f=25&p=526225#p526225
https://learn.adafruit.com/introducing-the-adafruit-bluefruit-le-uart-friend?view=all
https://github.com/01org/corelibs-arduino101/tree/master/libraries/CurieBLE
https://learn.adafruit.com/adafruit-feather-32u4-bluefruit-le/ble-services
http://www.freebsddiary.org/APC/usb_hid_usages.php
Hello, great post!
Have you ever used the Bluno Beetle as an input to Unity3d? I am currently trying to do that but the Unity application on my computer isn’t responding to it. I know the code works as I have used it with an Arduino Uno and I know that the Beetle is transmitting data as the Serial Monitor on the Arduino IDE shows the data received from the Beetle.